type
status
date
slug
summary
tags
category
icon
password
AI summary
链表的介绍
概念
链表是有序的列表,但是它在
内存中是存储
如下
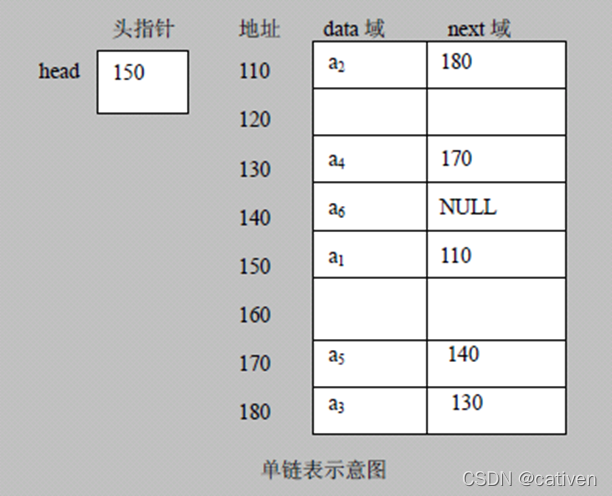
小结:
- 链表是以节点的方式来存储,是链式存储
- 每个节点包含 data 域, next 域:指向下一个节点.
- 如图:发现链表的各个节点不一定是连续存储.
- 链表分带头节点的链表和没有头节点的链表,根据实际的需求来确定
单链表的应用实例
案例
使用带head头的单向链表实现 –
水浒英雄排行榜
管理完成对英雄人物的
增删改查操作
, 注: 删除和修改,查找
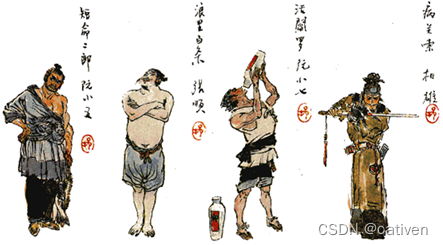
解题思路
1、增加
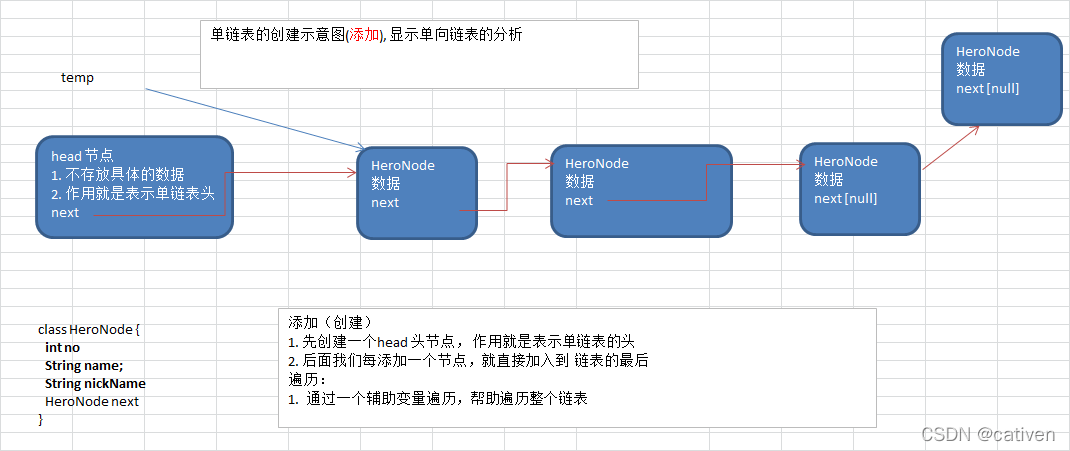
2、顺序添加(按照no由小到大排序)
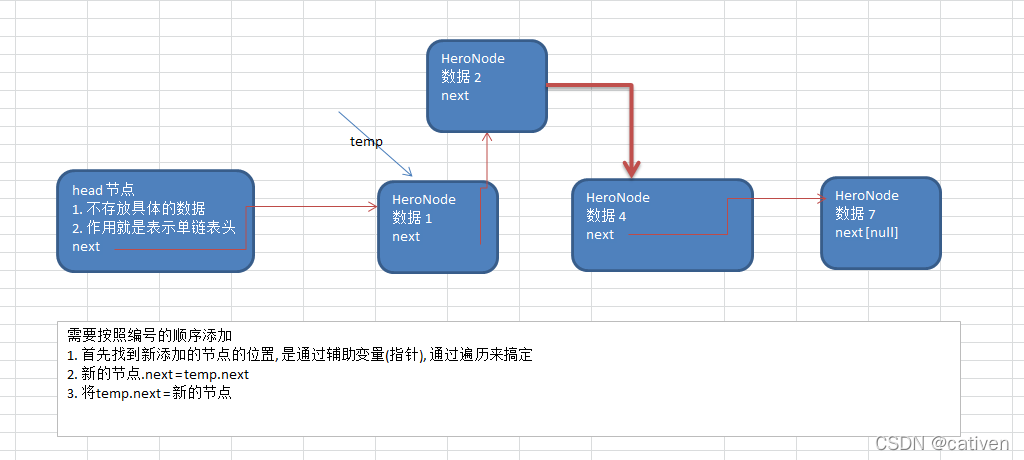
3、修改和删除节点(原理差不多)
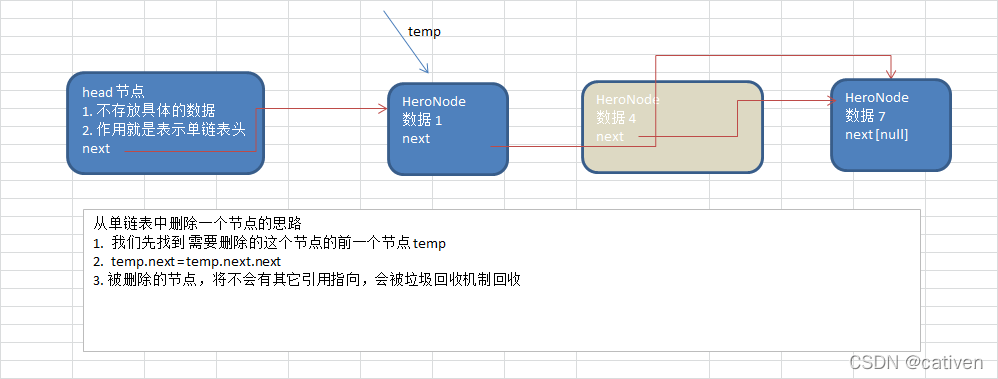
代码实现
1、新建英雄类,定义编号,名字,绰号,下一个节点的基本属性
2、新建LinkedList类,实现节点的增删改查方法
3、代码整合,整合英雄类和linkedList类
结果
1、add 添加节点
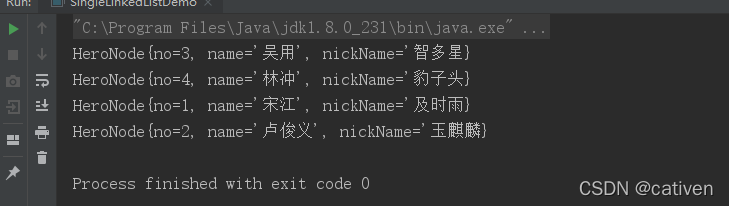
2、addOrderly(有序)添加节点
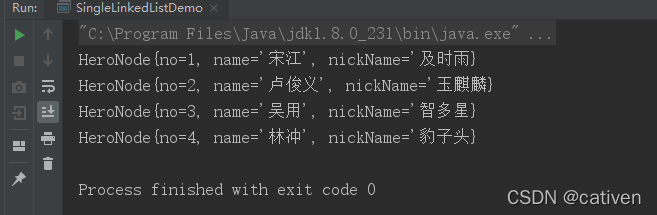
3、update 更新节点
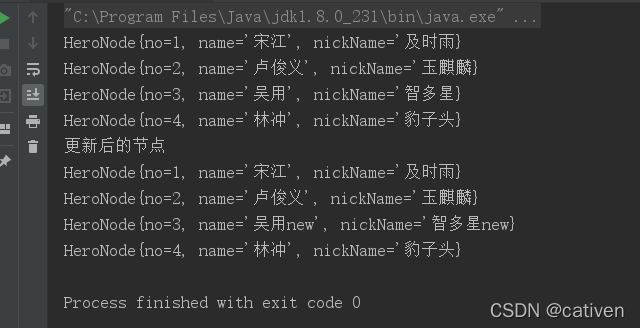
4、delete 删除节点
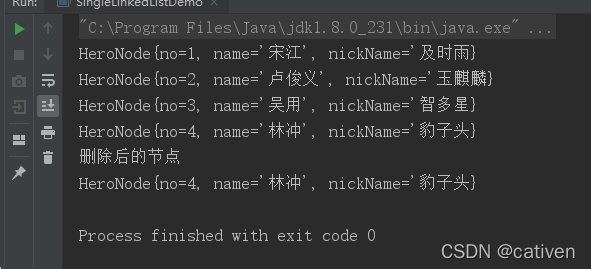
如有不正确的,还请指出!!!
- 作者:IT小舟
- 链接:https://www.codezhou.top/article/%E5%8D%95%E5%90%91%E9%93%BE%E8%A1%A8%E7%9A%84java%E5%AE%9E%E7%8E%B0%20%E8%AF%A6%E7%BB%86%E8%AE%B2%E8%A7%A3
- 声明:本文采用 CC BY-NC-SA 4.0 许可协议,转载请注明出处。